Interfacing 🧱Lego BOOST with a computer
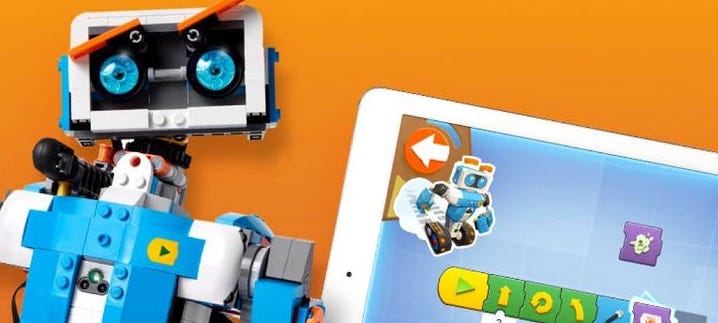
It turns out LEGO® Boost is quite a cool low-fi robotics thingy to play around with. Kids off the age of 7 could easily gain first experiences. Even for young grown ups there are quite some nice things to build and discover (admittedly those might get into Lego Mindstorms the earlier the better).
There is a lot of useful information about Boost on the Interwebs. In short you get a couple of motors and a color and distance sensor all connectable to a rather large central brick called Move Hub. Programming, audio I/O etc. are done through an application on your iPad or compatible tablet.
Tempting enough the connection between the Move Hub and your device is established using Bluetooth. Cool enough the protocol used is open sourced by Lego (https://lego.github.io/lego-ble-wireless-protocol-docs/index.html) which makes twiddling with that easier as expected.
There seem to be some libraries doing the job so let's prepare a proper Python environment with the following boost.yml:
name: boost
channels:
- conda-forge
- defaults
dependencies:
- python=3.9
- jupyter
- pip
- pip:
- Pillow
- bleak
- pylgbst
Make sure to activate the newly created environment and start
> jupyter notebook
A quick scribbled cell will test if scanning for Bluetooth devices works:
import asyncio
from bleak import BleakScanner
devices = await BleakScanner.discover()
for d in devices:
print(d)
Hint: The Jupyter kernel might break when executing this cell. On Mac OS X it turns out that this is a security thing. What I did to fix it was to add my Terminal app (in my case iTerm2.app) to System Preferences -> Security & Privacy -> Bluetooth.
In my case this listed quite some devices in range:
479359C2-3DAA-4779-89AA-D0EA89A1DF14: Unknown
D8C748DE-C6A1-47FF-860C-B16824F51CA1: Unknown
44F09E76-EDC7-4DDE-990A-6BEC539DAB15: iPad
758D98A2-78C1-4A90-ABF8-ECBD41143654: Unknown
2EAAAD5B-84D5-4D0D-A995-3597FB451AD5: iPhone
2A8F7974-8259-4854-8FB2-D7F3CE97308B: Unknown
453BC37A-1C6D-40F1-B16A-12A5F0A6C751: Unknown
F17ECFA0-00B7-4F79-BAA9-A0A11C403ECF: Unknown
BFD8C791-50EE-459C-A77C-EFD7914466EF: Apple Watch
EA37CF78-AACD-3B0E-BFE4-D4C9D8DACADF: Unknown
2D15766E-F5A3-4267-B825-1F19485DF24B: Unknown
6EE0045C-1279-4646-B422-CBABBF32CC0A: Move Hub
Obviously there is some general Bluetooth connectivity let's see what pylgbst adds to the soup 🍜:
import time
from time import sleep
from pylgbst import *
from pylgbst.hub import MoveHub
from pylgbst.peripherals import EncodedMotor, TiltSensor, Current, Voltage, COLORS, COLOR_BLACK
from pylgbst import get_connection_bleak
conn = get_connection_bleak(hub_mac='6EE0045C-1079-4646-B422-CEABBF32CC0A')
movehub = MoveHub(conn)
for device in movehub.peripherals:
print(device)
movehub.disconnect()
This snippet should connect to the Move Hub with this dedicated ID and list its peripherals. If you are operating under Windows I assume the real mac address needs to be provided. For security reasons Mac OS X prevents showing the mac addresses and uses the UUID instead.
Now let's try to move the motor. Switch on the Move Hub and execute this cell:
conn = get_connection_bleak(hub_mac='6EE0045C-1279-4646-B422-CBABBF32CC0A')
movehub = MoveHub(conn)
for level in range(0, 101, 10):
level /= 100.0
print("Speed level: ", level * 100)
movehub.motor_A.timed(0.2, level)
movehub.motor_B.timed(0.2, -level)
movehub.motor_AB.timed(1.5, -0.2, 0.2)
movehub.motor_AB.timed(0.5, 1)
movehub.motor_AB.timed(0.5, -1)
movehub.disconnect()
Useful Links
https://github.com/hbldh/bleak/issues/438
https://awesomeopensource.com/project/undera/pylgbst
* Header picture courtesy of Lego, 2021